mirror of
https://github.com/blawar/ooot.git
synced 2024-07-04 18:13:37 +00:00
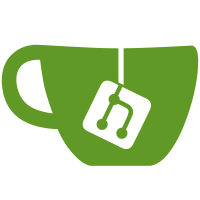
* Updated config file * Added missing files * Temporarily removed asm_processor changes. * git subrepo pull --force tools/ZAPD subrepo: subdir: "tools/ZAPD" merged: "96ffc1e62" upstream: origin: "https://github.com/zeldaret/ZAPD.git" branch: "master" commit: "96ffc1e62" git-subrepo: version: "0.4.3" origin: "???" commit: "???" * git subrepo pull --force tools/ZAPD subrepo: subdir: "tools/ZAPD" merged: "179af7d11" upstream: origin: "https://github.com/zeldaret/ZAPD.git" branch: "master" commit: "179af7d11" git-subrepo: version: "0.4.3" origin: "???" commit: "???" * Cleanup and fixes. * git subrepo pull --force tools/ZAPD subrepo: subdir: "tools/ZAPD" merged: "50ad2fe78" upstream: origin: "https://github.com/zeldaret/ZAPD.git" branch: "master" commit: "50ad2fe78" git-subrepo: version: "0.4.3" origin: "???" commit: "???" * Makefile fix * git subrepo pull --force tools/ZAPD subrepo: subdir: "tools/ZAPD" merged: "b9120803e" upstream: origin: "https://github.com/zeldaret/ZAPD.git" branch: "master" commit: "b9120803e" git-subrepo: version: "0.4.3" origin: "???" commit: "???" Co-authored-by: Jack Walker <7463599+Jack-Walker@users.noreply.github.com>
56 lines
2.1 KiB
Python
Executable File
56 lines
2.1 KiB
Python
Executable File
#!/usr/bin/env python3
|
|
|
|
import argparse
|
|
import os
|
|
from shutil import copyfile
|
|
from multiprocessing import Pool
|
|
from multiprocessing import cpu_count
|
|
|
|
def Extract(xmlPath, outputPath, outputSourcePath):
|
|
ExtractFile(xmlPath, outputPath, outputSourcePath, 1, 0)
|
|
|
|
def ExtractScene(xmlPath, outputPath, outputSourcePath):
|
|
ExtractFile(xmlPath, outputPath, outputSourcePath, 1, 1)
|
|
|
|
def ExtractFile(xmlPath, outputPath, outputSourcePath, genSrcFile, incFilePrefix):
|
|
execStr = "tools/ZAPD/ZAPD.out e -eh -i %s -b baserom/ -o %s -osf %s -gsf %i -ifp %i -rconf tools/ZAPDConfigs/MqDbg/Config.xml" % (xmlPath, outputPath, outputSourcePath, genSrcFile, incFilePrefix)
|
|
|
|
print(execStr)
|
|
os.system(execStr)
|
|
|
|
def ExtractFunc(fullPath):
|
|
outPath = ("assets/" + fullPath.split("assets/xml/")[1]).split(".xml")[0]
|
|
outSourcePath = ("assets/" + fullPath.split("assets/xml/")[1]).split(".xml")[0]
|
|
|
|
if (fullPath.startswith("assets/xml/scenes/")):
|
|
ExtractScene(fullPath, outPath, outSourcePath)
|
|
else:
|
|
Extract(fullPath, outPath, outSourcePath)
|
|
|
|
def main():
|
|
parser = argparse.ArgumentParser(description="baserom asset extractor")
|
|
parser.add_argument("-s", "--single", help="asset path relative to assets/, e.g. objects/gameplay_keep")
|
|
args = parser.parse_args()
|
|
|
|
asset_path = args.single
|
|
if asset_path is not None:
|
|
if asset_path.endswith("/"):
|
|
asset_path = asset_path[0:-1]
|
|
Extract(f"assets/xml/{asset_path}.xml", f"assets/{asset_path}/", f"assets/{asset_path}/")
|
|
else:
|
|
xmlFiles = []
|
|
for currentPath, folders, files in os.walk("assets"):
|
|
for file in files:
|
|
fullPath = os.path.join(currentPath, file)
|
|
if file.endswith(".xml") and currentPath.startswith("assets/xml/"):
|
|
outPath = ("assets/" + fullPath.split("assets/xml/")[1]).split(".xml")[0]
|
|
xmlFiles.append(fullPath)
|
|
|
|
numCores = cpu_count()
|
|
print("Extracting assets with " + str(numCores) + " CPU cores.")
|
|
p = Pool(numCores)
|
|
p.map(ExtractFunc, xmlFiles)
|
|
|
|
if __name__ == "__main__":
|
|
main()
|